Hosting a thin static blog on a platform like GitHub Pages has numerous advantages but also takes away some interactivity. Fortunately, Giscus exists and offers a way to embed user comments on static sites.
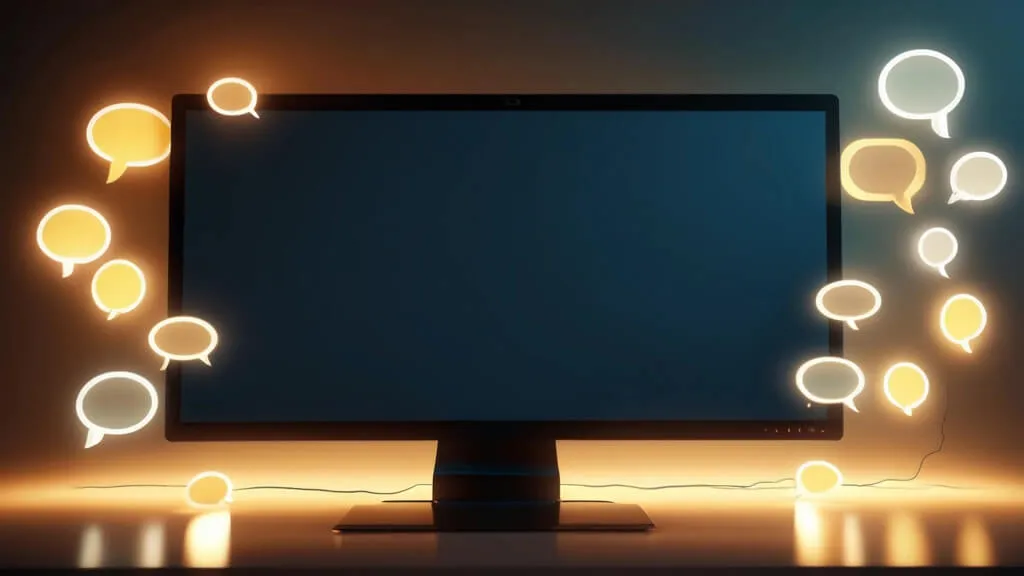
How Giscus works
Giscus uses the GitHub API to read and store comments made by GitHub users in the Discussions
associated with a repository.
Embed the Giscus client-side script bundle on your site, configure it with the correct repository URL, and users can view and write comments (when logged into GitHub).
The approach is serverless, as the comments are stored on GitHub and dynamically loaded from there on the client side, hence perfect for a static blog.
Setting up Giscus
Giscus can be set up easily on giscus.app, but I will outline the process shortly still.
Prequisites
Prequisites to get Giscus working are
- the repository is public
- the Giscus app is installed
- the Discussions feature is turned on for your repository
If any of these conditions cannot be fulfilled for any reason, unfortunately, Giscus cannot be integrated.
Configuring Giscus
Next, configuring Giscus is necessary. In most cases, the preselected defaults are suitable, and you should only modify them if you have a specific reason and know what you are doing. Don’t worry too much about making the wrong choices; you can always adjust the configuration later on.
However, you need to
- select the right language for the UI
- specify the GitHub repository you want to connect, typically the repository containing your statically hosted site on GitHub Pages
- create and set an
Announcement
type discussion on GitHub if you want to ensure nobody can create random comments directly on GitHub - define the color scheme
After configuring the settings, Giscus provides you with a generated <script>
tag, which you will need in the next steps.
Simple script tag
You should now have a script tag that looks like this:
<script
src="https://giscus.app/client.js"
data-repo="[ENTER REPO HERE]"
data-repo-id="[ENTER REPO ID HERE]"
data-category="[ENTER CATEGORY NAME HERE]"
data-category-id="[ENTER CATEGORY ID HERE]"
data-mapping="pathname"
data-strict="0"
data-reactions-enabled="1"
data-emit-metadata="0"
data-input-position="bottom"
data-theme="preferred_color_scheme"
data-lang="en"
crossorigin="anonymous"
async
></script>
Simply add that to the source code of the site. For instance, if you’re using Nordlys (the theme of this website) and want to enable comments on posts, navigate to src/layouts/PostLayout.astro
and paste it into the desired location where you want the comments to appear, perhaps underneath the <Content>
.
src/layouts/PostLayout.astro
<article>
<Content />
</article>
+ <script src="https://giscus.app/client.js"
+ data-repo="[ENTER REPO HERE]"
+ data-repo-id="[ENTER REPO ID HERE]"
+ data-category="[ENTER CATEGORY NAME HERE]"
+ data-category-id="[ENTER CATEGORY ID HERE]"
+ ...
+ </script>
<AdjacentPostsBar {post} slot="bottom" />
And it’s done! You have successfully integrated comments on your site!